狂神说POI及EasyExcel
EXCEL基本写操作
excel中的对象
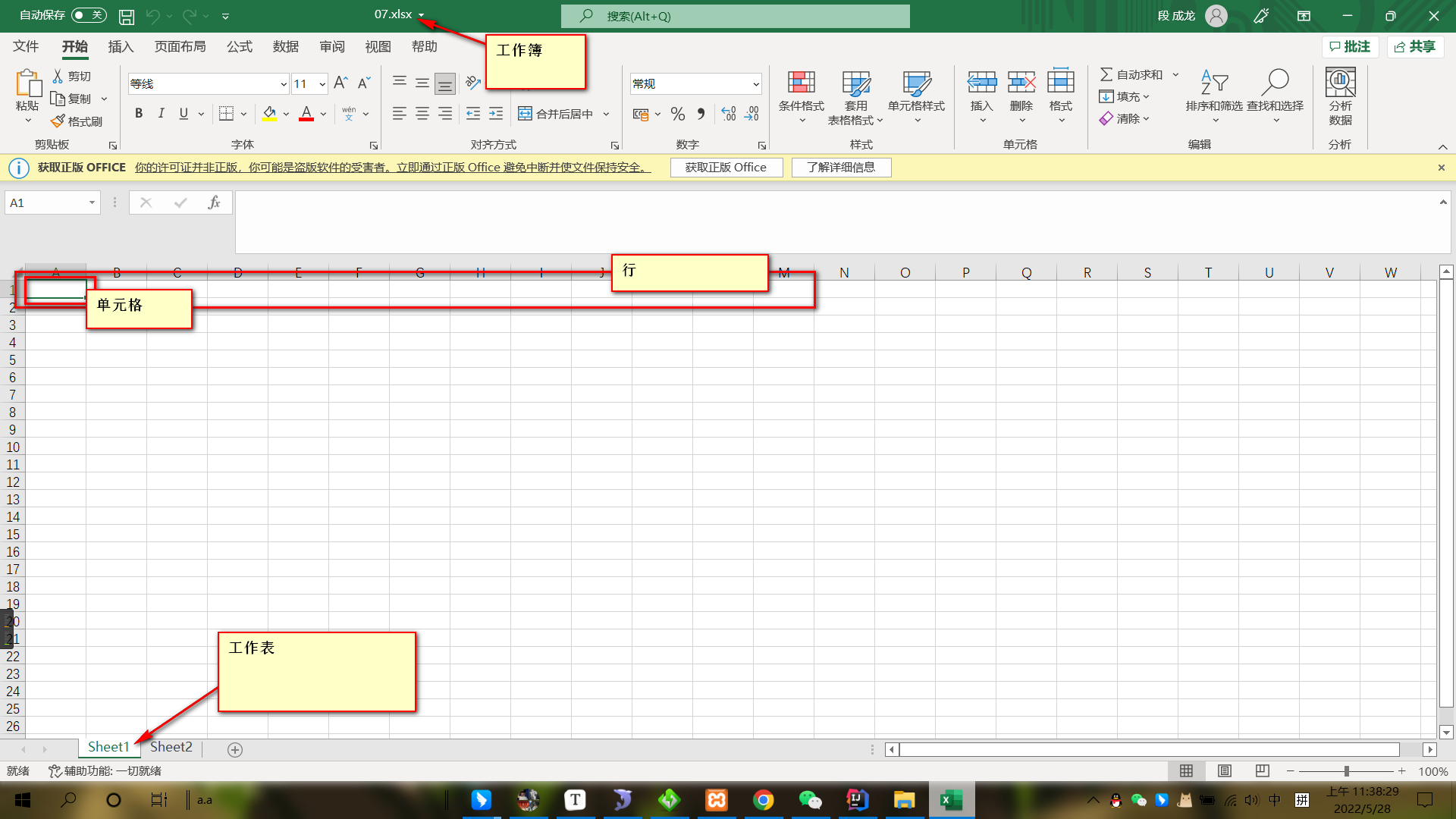
对象的对应实现类
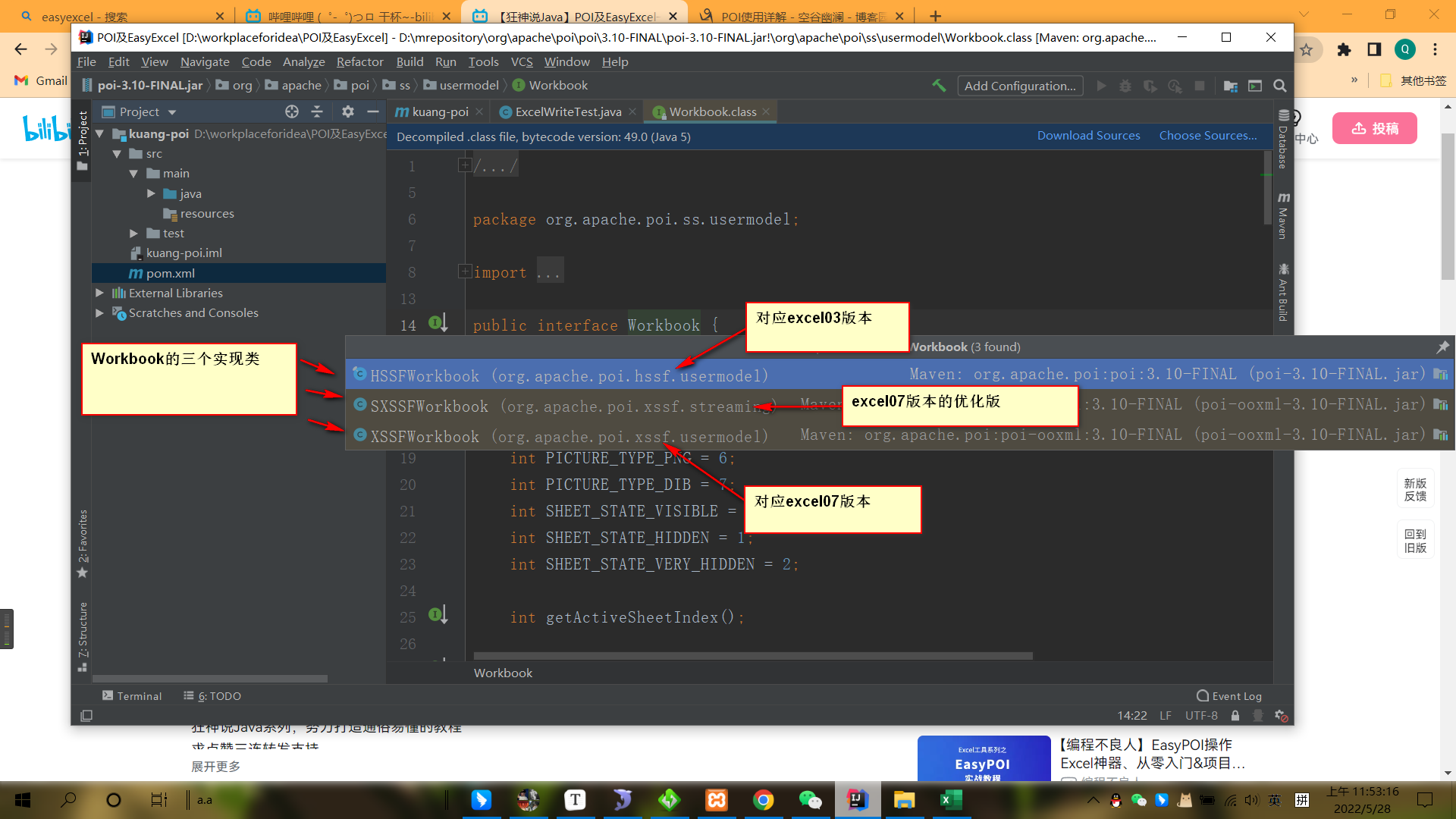
导入maven依赖
1 | <!--导入依赖--> |
joda-time是一个常用的时间处理类库
大数据量的写入
-
@Test public void testWrite03BigData() throws IOException { // 时间 long begin = System.currentTimeMillis(); // 创建一个工作簿 Workbook workbook = new HSSFWorkbook(); // 创建表 Sheet sheet = workbook.createSheet(); // 写入数据 for (int rowNum = 0; rowNum < 65536; rowNum++){ Row row = sheet.createRow(rowNum); for (int cellNum = 0; cellNum < 10; cellNum++) { Cell cell = row.createCell(cellNum); cell.setCellValue(cellNum); } } System.out.println("over"); // 开启IO流 FileOutputStream fileOutputStream = new FileOutputStream(PATH + "testWrite03BigData.xls"); // 写出数据 workbook.write(fileOutputStream); // 关闭IO流 fileOutputStream.close(); long end = System.currentTimeMillis(); System.out.print((double) (end - begin) / 1000); }
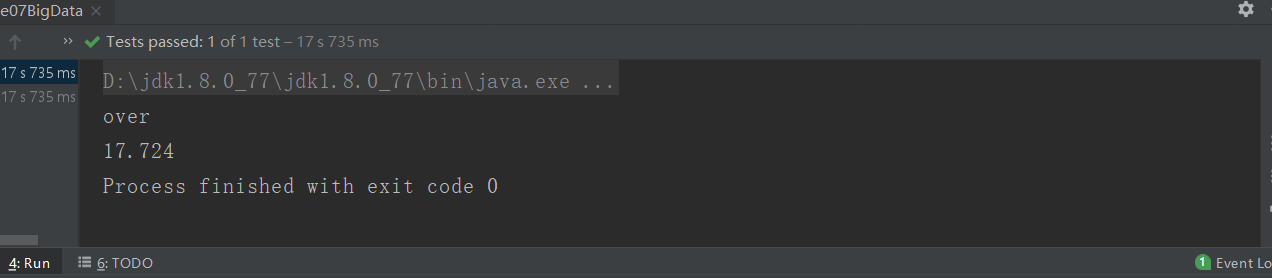 > xlsx写入速度较慢,数据无上限1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35

> xls只能写入65536行数据,否则报错
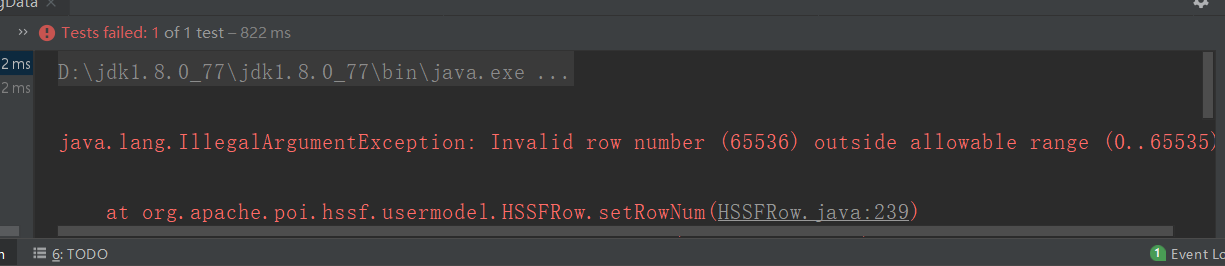
2. ```java
@Test
public void testWrite07BigData() throws IOException {
// 时间
long begin = System.currentTimeMillis();
// 创建一个工作簿
Workbook workbook = new XSSFWorkbook();
// 创建表
Sheet sheet = workbook.createSheet();
// 写入数据
for (int rowNum = 0; rowNum < 65537; rowNum++){
Row row = sheet.createRow(rowNum);
for (int cellNum = 0; cellNum < 10; cellNum++) {
Cell cell = row.createCell(cellNum);
cell.setCellValue(cellNum);
}
}
System.out.println("over");
// 开启IO流
FileOutputStream fileOutputStream = new FileOutputStream(PATH + "testWrite07BigData.xlsx");
// 写出数据
workbook.write(fileOutputStream);
// 关闭IO流
fileOutputStream.close();
long end = System.currentTimeMillis();
System.out.print((double) (end - begin) / 1000);
} -
@Test public void testWrite07BigDataS() throws IOException { // 时间 long begin = System.currentTimeMillis(); // 创建一个工作簿 Workbook workbook = new SXSSFWorkbook(); // 创建表 Sheet sheet = workbook.createSheet(); // 写入数据 for (int rowNum = 0; rowNum < 65537; rowNum++){ Row row = sheet.createRow(rowNum); for (int cellNum = 0; cellNum < 10; cellNum++) { Cell cell = row.createCell(cellNum); cell.setCellValue(cellNum); } } System.out.println("over"); // 开启IO流 FileOutputStream fileOutputStream = new FileOutputStream(PATH + "testWrite07BigDataS.xlsx"); // 写出数据 workbook.write(fileOutputStream); // 关闭IO流 fileOutputStream.close(); // 清楚加速版中运用内存缓冲带来的临时文件 ((SXSSFWorkbook)workbook).dispose(); long end = System.currentTimeMillis(); System.out.print((double) (end - begin) / 1000); }
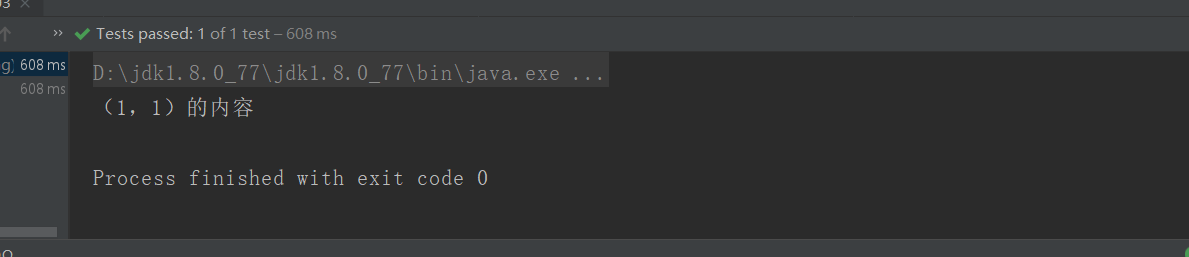1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
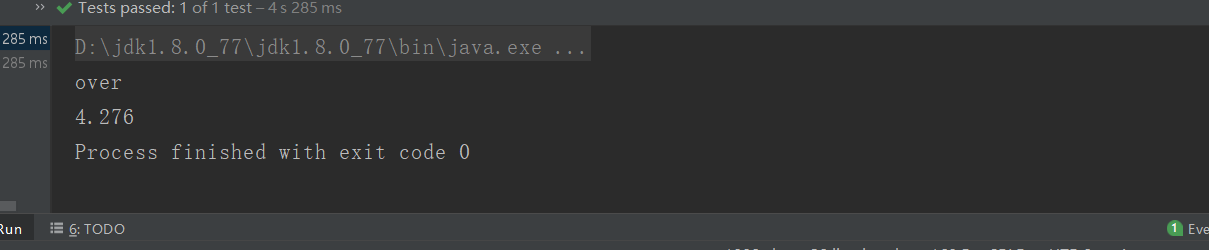
> xlsx加速版,SXSSFWorkbook类,结束时需要清除内存缓存。
# Excel基本读取及注意
1. ```java
@Test
public void testRead03() throws Exception {
// 获取文件流
FileInputStream fileInputStream = new FileInputStream(PATH + "\\kuang-poi狂神观众统计表03.xls");
// 1.创建一个工作簿
HSSFWorkbook workbook = new HSSFWorkbook(fileInputStream);
// 2.得到表
Sheet sheet = workbook.getSheetAt(0);
// 3.得到行
Row row = sheet.getRow(0);
// 4.得到列
Cell cell = row.getCell(0);
System.out.println(cell.getStringCellValue());
// 关闭流
fileInputStream.close();
} -
@Test public void testRead07() throws Exception { // 获取文件流 FileInputStream fileInputStream = new FileInputStream(PATH + "\\kuang-poi狂神观众统计表07.xlsx"); // 1.创建一个工作簿 Workbook workbook = new XSSFWorkbook(fileInputStream); // 2.得到表 Sheet sheet = workbook.getSheetAt(0); // 3.得到行 Row row = sheet.getRow(0); // 4.得到列 Cell cell = row.getCell(0); Cell cell1 = row.getCell(1); System.out.println(cell.getStringCellValue()); System.out.println(cell1.getNumericCellValue()); // 关闭流 fileInputStream.close(); }
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
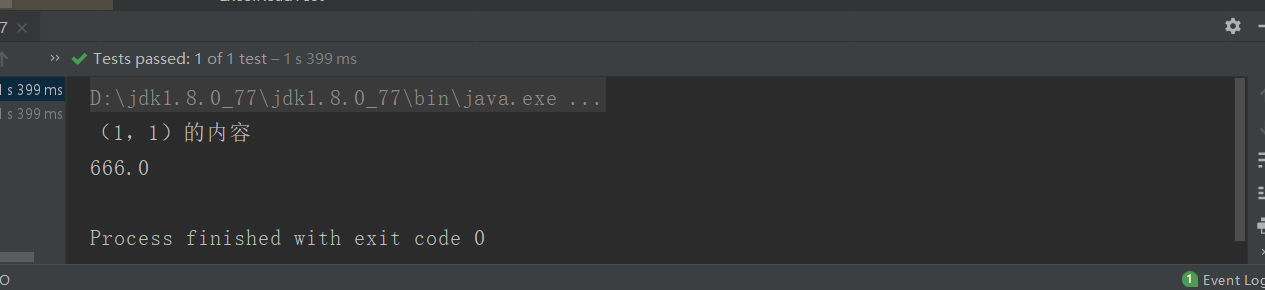
# 读取不同类型数据,批量读取,可以直接用
```java
@Test
public void testCellType() throws Exception {
// 获取文件流
FileInputStream fileInputStream = new FileInputStream(PATH + "\\kuang-poi狂神观众统计表07.xlsx");
// 1.创建一个工作簿
Workbook workbook = new XSSFWorkbook(fileInputStream);
// 2.得到表
Sheet sheet = workbook.getSheetAt(0);
// 获取表中的内容
// 获取行数
int rowCount = sheet.getPhysicalNumberOfRows();
for (int rowNum = 0; rowNum < rowCount; rowNum++) {
Row rowData = sheet.getRow(rowNum);
if (rowData != null) {
// 获取每行列数
int cellCount = rowData.getPhysicalNumberOfCells();
// 遍历单元格
for (int cellNum = 0; cellNum < cellCount; cellNum++) {
// 读取单元格
Cell cell = rowData.getCell(cellNum);
String cellValue = "";
// 匹配单元格数据类型
if (cell != null) {
int cellType = cell.getCellType();
switch (cellType) {
case Cell.CELL_TYPE_STRING: // 字符串
System.out.print("[String]");
cellValue = cell.getStringCellValue();
break;
case Cell.CELL_TYPE_BOOLEAN: // 布尔值
System.out.print("[BOOLEAN]");
cellValue = String.valueOf(cell.getBooleanCellValue());
break;
case Cell.CELL_TYPE_BLANK: // 空
System.out.print("[BLANK]");
break;
case Cell.CELL_TYPE_NUMERIC: // 数字(日期,普通数字)
System.out.print("[NUMERIC]");
if (DateUtil.isCellDateFormatted(cell)){ // 日期
System.out.print("[日期]");
Date date = cell.getDateCellValue();
cellValue = new DateTime(date).toString("yyyy-MM-dd");
} else{
System.out.print("[转换为字符串输出]");
cell.setCellType(Cell.CELL_TYPE_STRING);
cellValue = cell.toString();
}
break;
case Cell.CELL_TYPE_ERROR: // 错误
System.out.print("[数据类型错误]");
break;
}
System.out.println(cellValue);
}
}
}
fileInputStream.close();
}
}
了解-计算公式
1 |
|
easyExcel操作
导入依赖
1 | <!-- easyExcel --> |
固定套路
1.写入根据固定类格式进行写入
2.读取根据监听器设置的规则进行读取
本博客所有文章除特别声明外,均采用 CC BY-NC-SA 4.0 许可协议。转载请注明来自 小蜗!
评论
ValineDisqus