mabatis-plus
CRUD扩展
不同的主键策略测试
主键自增
-
实体类上添加代码:
1
-
数据库字段设置自增
其他主键策略
1 | public enum IdType { |
乐观锁处理讲解
乐观锁,一般不锁
悲观锁,一直锁着
-
给数据库中添加version字段
-
实体类加对应的字段
-
注册组件
1
2
3
4
5
6
7
8
9
10
11
12
13
//配置类
public class MyBatisPlusConfig {
// 注册乐观锁插件
/**
* 旧版
*/
public OptimisticLockerInterceptor optimisticLockerInterceptor() {
return new OptimisticLockerInterceptor();
}
}
多条件查询
1 | //tiao条件查询map |
分页查询操作
mybatis_plus其实内置了分页插件
-
配置拦截器组件即可
-
// 旧版 // 分页插件 @Bean public PaginationInterceptor paginationInterceptor() { return new PaginationInterceptor(); }
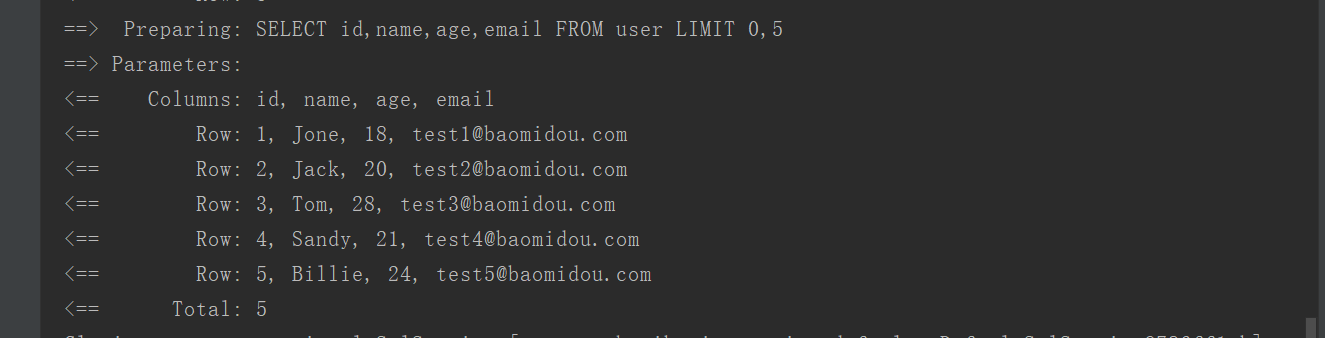1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
3. ```java
// 测试分页查询
@Test
public void testPage() {
// IPage接口实现类
// 参数一:当前页
// 参数二:页面大小
Page<User> page = new Page<>(1,5);
// IPage<T> selectPage(IPage<T> var1, @Param("ew") Wrapper<T> var2);
userMapper.selectPage(page, null);
page.getRecords().forEach(System.out::println);
// 获取数据总量
System.out.println(page.getTotal());
}
删除操作
根据ID删除记录
1 | // 测试删除 |
批量删除
1 | // 测试通过ID批量删除 |
根据条件删除
1 | // 通过map删除 |
条件构造器
wrapper,替代复杂sql语句
-
@Test void contextLoads() { //查询name和email不为空的用户,年龄大于等于12 QueryWrapper<User> wrapper = new QueryWrapper<>(); wrapper.isNotNull("name") .isNotNull("email") .ge("age",12); userMapper.selectList(wrapper); }
1
2
3
4
5
6
7
8
9
10
2. ```java
@Test
void test2(){
// 查询名字狂神说
QueryWrapper<User> wrapper = new QueryWrapper<>();
wrapper.eq("name", "Tom");
User user = userMapper.selectOne(wrapper);
System.out.println(user);
} -
@Test void test3(){ // 查询年龄在20~30之间的用户 QueryWrapper<User> wrapper = new QueryWrapper<>(); wrapper.between("age", 20, 30); Integer count = userMapper.selectCount(wrapper); System.out.println(count); }
1
2
3
4
5
6
7
8
9
10
11
12
4. ```java
// 模糊查询
@Test
void test4(){
// 邮箱t开头,名字中不含字母e的用户
QueryWrapper<User> wrapper = new QueryWrapper<>();
wrapper.notLike("name", "e")
.likeRight("email", "t");
List<Map<String,Object>> maps = userMapper.selectMaps(wrapper);
maps.forEach(System.out::println);
}
代码生成器
-
3.0.7版本移除 对 mybatis-plus-generator 包的依赖,自己按需引入,尽在还需要导入模板依赖,
org.apache.velocity velocity-engine-core 2.0 -
package com.kuang; import com.baomidou.mybatisplus.annotation.DbType; import com.baomidou.mybatisplus.annotation.IdType; import com.baomidou.mybatisplus.generator.AutoGenerator; import com.baomidou.mybatisplus.generator.config.DataSourceConfig; import com.baomidou.mybatisplus.generator.config.GlobalConfig; import com.baomidou.mybatisplus.generator.config.PackageConfig; import com.baomidou.mybatisplus.generator.config.StrategyConfig; import com.baomidou.mybatisplus.generator.config.rules.DateType; import com.baomidou.mybatisplus.generator.config.rules.NamingStrategy; /** * TODO * * @ClassName KuangCode * @Author Alfa * @Data 2022/5/26 14:27 * @Version 1.0 **/ public class KuangCode { public static void main(String[] args) { // 构建代码自动生成器对象 AutoGenerator mpg = new AutoGenerator(); // 配置策略 // 1.全局配置 GlobalConfig gc = new GlobalConfig(); String projectPath= System.getProperty("user.dir"); gc.setOutputDir(projectPath + "/src/main/java"); gc.setAuthor("alfa"); gc.setOpen(false); gc.setFileOverride(false); // 是否覆盖 gc.setServiceName("%sService"); // 去service的i前缀 gc.setIdType(IdType.ID_WORKER); gc.setDateType(DateType.ONLY_DATE); gc.setSwagger2(true); mpg.setGlobalConfig(gc); //2.设置数据源 DataSourceConfig dsc = new DataSourceConfig(); dsc.setUrl("jdbc:mysql://localhost:3306/mybatis_plus?useSSL=false&useUnicode=true&characterEncoding=utf8"); dsc.setDriverName("com.mysql.cj.jdbc.Driver"); dsc.setUsername("root"); dsc.setPassword(""); dsc.setDbType(DbType.MYSQL); mpg.setDataSource(dsc); // 3。包的配置 PackageConfig pc = new PackageConfig(); pc.setModuleName("blog"); pc.setParent("com.kuang"); pc.setEntity("pojo"); pc.setMapper("mapper"); pc.setService("service"); pc.setController("controller"); mpg.setPackageInfo(pc); //5.策略配置 StrategyConfig strategy = new StrategyConfig(); strategy.setInclude("user"); strategy.setNaming(NamingStrategy.underline_to_camel); strategy.setColumnNaming(NamingStrategy.underline_to_camel); strategy.setEntityLombokModel(true); //自动生成lombok注解 strategy.setLogicDeleteFieldName("deleted"); //自动填充配置 // TableFill gmtCreate = new TableFill("gmt_create", FieldFill.INSERT); // TableFill gmtModified = new TableFill("gmt_modified", FieldFill.INSERT_UPDATE); // ArrayList<TableFill> tableFills = new ArrayList<>(); // tableFills.add(gmtCreate); // tableFills.add(gmtModified); // strategy.setTableFillList(tableFills); // 乐观锁 // strategy.setVersionFieldName("version"); // 驼峰命名 strategy.setRestControllerStyle(true); strategy.setControllerMappingHyphenStyle(true); // localhost:8080/hello_iu_2 mpg.setStrategy(strategy); mpg.execute(); } }
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
3. <img src="https://s2.loli.net/2022/05/27/6K8OTpCWrlkezSJ.png" style="zoom: 50%;" />
# MybatisPlus 代码生成器 出现 实体类的@ApiModel 注解报错 解决方法
> 问题
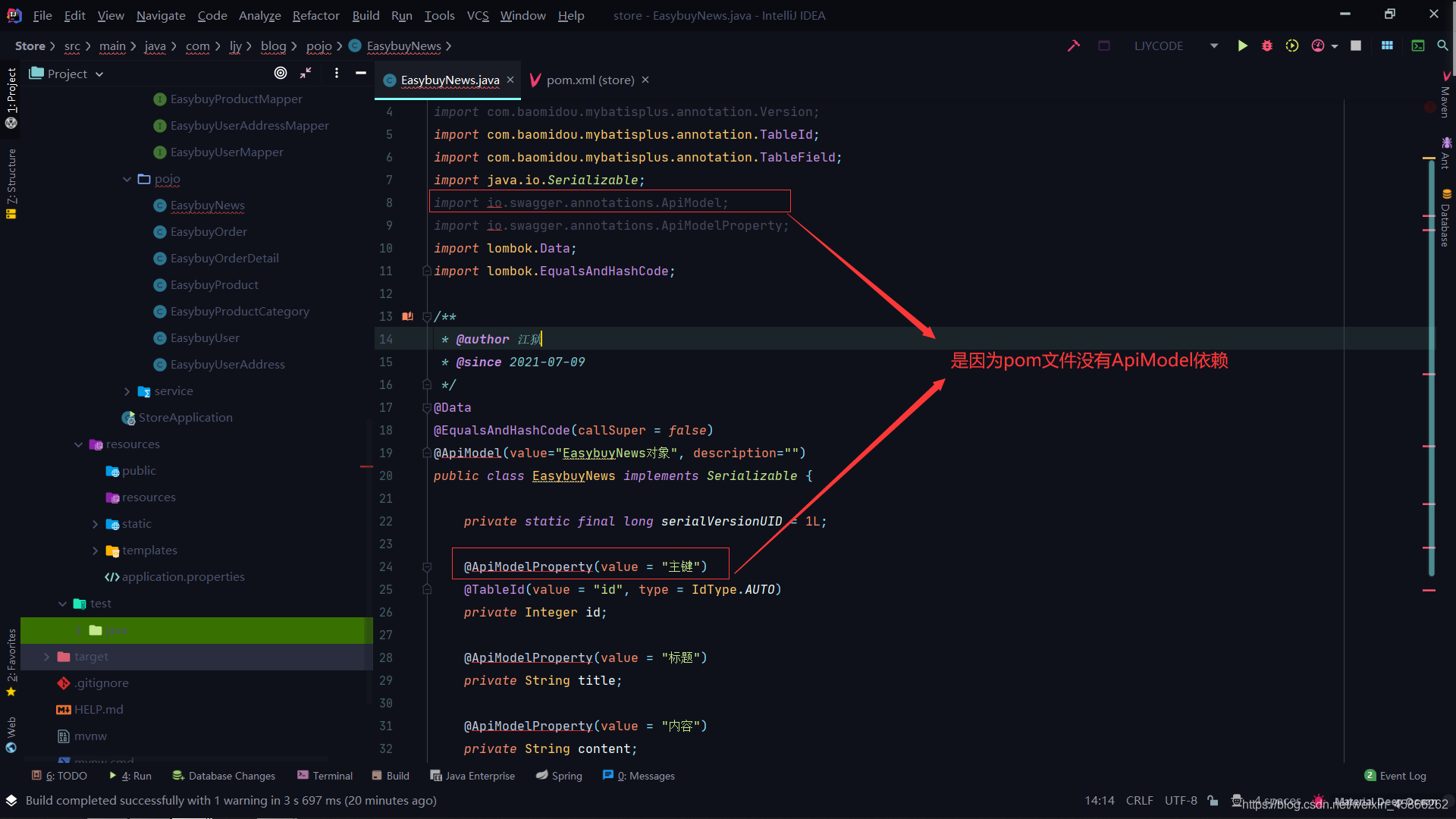
> 解决问题
在pom.[xml](https://so.csdn.net/so/search?q=xml&spm=1001.2101.3001.7020) 文件中添加 ApiModel 依赖 就不会报错了
```XML
<!--配置ApiModel在实体类中不生效-->
<dependency>
<groupId>com.spring4all</groupId>
<artifactId>spring-boot-starter-swagger</artifactId>
<version>1.5.1.RELEASE</version>
</dependency>
Mybatis-plus最新代码生成器(3.5.1+)的使用
解决mybatis-plus代码生成器在idea中的module生成代码,但是生成到外面的project
本博客所有文章除特别声明外,均采用 CC BY-NC-SA 4.0 许可协议。转载请注明来自 小蜗!
评论
ValineDisqus